Django change button status
Hi,
I search through internet and review many apps but still don't have answer maybe on this forum someone help me.
I try to create changing button after user join to Goal.
I have work buttons, but i don't know how to change this in template.
I try to do this with boolean field, when user click "Join", boolean field change to True, and then in templates:
{% if goal.joined %} <Delete join> {% else %} <join to goal> {% endif %}
For now user can click many times on button and create many "join" objects in one goal.
Now i know that i need to create variable in view that checks if the current user has joined the goal and add it to the context. But I don't know how. Im still newby.
class Goal(models.Model, Activity): title = models.CharField(max_length=255, verbose_name='Tytuł') image = models.ImageField(upload_to='goals', verbose_name='Tło') body = HTMLField(verbose_name='Treść') tags = TaggableManager() created_at = models.DateTimeField(auto_now_add=True) author = models.ForeignKey(User, on_delete=models.CASCADE) slug = AutoSlugField(populate_from='title') def __str__(self): return self.title class Meta: verbose_name = 'Cele' ordering = ['-created_at'] def get_absolute_url(self): return reverse('goaldetail', args=[str(self.slug)]) @property def activity_actor_attr(self): return self.author def add_user_to_list_of_attendees(self, user): registration = Joined.objects.create(user = user, goal = self, created_at = timezone.now()) def remove_user_from_list_of_attendees(self, user): registration = Joined.objects.get(user = user, goal = self) registration.delete() class Joined(models.Model, Activity): goal = models.ForeignKey(Goal, on_delete=models.CASCADE, related_name='joined') user = models.ForeignKey(User, on_delete=models.CASCADE, related_name='joined_users') created_at = models.DateTimeField(auto_now_add=True) joined = models.BooleanField(default=False) def save(self, *args, **kwargs): if self.id is None and self.created_at is None: self.created_at = datetime.datetime.now() self.joined = True super(Joined, self).save(*args, **kwargs) @property def activity_actor_attr(self): return self.user
Views:
def joined_add(request, pk): this_goal = Goal.objects.get(pk=pk) this_goal.add_user_to_list_of_attendees(user=request.user) return redirect('goaldetail', slug=this_goal.slug) def joined_delete(request, pk): this_goal = Goal.objects.get(pk=pk) this_goal.remove_user_from_list_of_attendees(request.user) return redirect('goaldetail', slug=this_goal.slug) def goaldetail(request, slug): goal = get_object_or_404(Goal, slug=slug) return render(request, 'goals/detail.html', {'goal': goal})
Path:
path('joined/<int:pk>', views.joined_add, name='joined_add'), path('joined-delete/<int:pk>', views.joined_delete, name='joined_delete'),
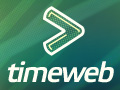
We recommend hosting TIMEWEB
Stable hosting, on which the social network EVILEG is located. For projects on Django we recommend VDS hosting.Do you like it? Share on social networks!
- Unknown akadamn
- Jan. 24, 2025, 5:14 p.m.
Qt - Test 001. Signals and slots
- Result:84points,
- Rating points4
- Unknown akadamn
- Jan. 24, 2025, 4:22 p.m.
Qt - Test 001. Signals and slots
- Result:42points,
- Rating points-8


Hello,
I think, You need to check user in joined_set in your case.
This is your custom template tag
The next step, check user in the set
Edit: Works! Thank you very much! Just change goal.joined_set.all to goal.joined.all. :D
ok. I see. You changed related name
Try this