How can I open SVG file through QT
I'm trying to develop flowchart diagram application using QT with C++. I'm planing to design basic shapes of flowchart, such as rectangle, ellipse,polygon and line item(arrow).In this code I designed rectangle and ellipse items and content of the QGraphicView saved properly.But problem is I can't open .SVG file properly. Program open rectangle shapes only. How can I open other shapes? such as ellipse,polygon and line item(arrow).
I'm new to this QT with C++ application development.So please help me to solve this problem.here with I attach sample .SVG file.
ReadSvg.cpp
#include "readsvg.h" #include <QPen> #include <QFile> #include <QMessageBox> #include <QDomDocument> #include <QStringList> ReadSVG::ReadSVG() { } QList<QGraphicsRectItem *> ReadSVG::getElements(const QString filename) { QList<QGraphicsRectItem *> rectList; // We declare in the stack a list of rectangles QDomDocument doc; // document object QFile file(filename); // Open our SVG file // If it did not open or could not transfer content to QDocDocument if (!file.open(QIODevice::ReadOnly) || !doc.setContent(&file)) return rectList; // then return the list, but empty // Look in the document for all objects with the tag g QDomNodeList gList = doc.elementsByTagName("g"); // We start to sort them out for (int i = 0; i < gList.size(); i++) { QDomNode gNode = gList.item(i); // Select the node from the list QDomElement rectangle = gNode.firstChildElement("rect"); // And we search in it for an element with the tag rect // If the resulting elements are not zero, then if (rectangle.isNull()){ continue; } else { // begin to form a rectangle QGraphicsRectItem *rect = new QGraphicsRectItem(); // This flag makes the object moveable, it will be required for verification rect->setFlag(QGraphicsItem::ItemIsMovable); // We take sizes from the rect tag QDomElement gElement = gNode.toElement(); rect->setRect(rectangle.attribute("x").toInt(), rectangle.attribute("y").toInt(), rectangle.attribute("width").toInt(), rectangle.attribute("height").toInt()); /* We take the parameters of the colors gNode from the node element yes yes yes ... it's from gNode, not from rectangle. These parameters are stored in the tag g */ QColor fillColor(gElement.attribute("fill", "#ffffff")); // fill color fillColor.setAlphaF(gElement.attribute("fill-opacity","0").toFloat()); rect->setBrush(QBrush(fillColor)); // as well as the color and thickness of the outline QColor strokeColor(gElement.attribute("stroke", "#000000")); strokeColor.setAlphaF(gElement.attribute("stroke-opacity").toFloat()); rect->setPen(QPen(strokeColor,gElement.attribute("stroke-width", "0").toInt())); rectList.append(rect); // add a rectangle to the list } } file.close(); return rectList; } QList<QGraphicsEllipseItem*> ReadSVG::getEllipses(const QString filename) { QList<QGraphicsEllipseItem *> ellipsesList; QDomDocument doc; QFile file(filename); if (!file.open(QIODevice::ReadOnly) || !doc.setContent(&file)) return ellipsesList; QDomNodeList gList = doc.elementsByTagName("g"); for (int i = 0; i < gList.size(); i++) { QDomNode gNode = gList.item(i); QDomElement ellipseElement = gNode.firstChildElement("ellipse"); if (ellipseElement.isNull()){ continue; } else { QGraphicsEllipseItem *ellipseItem = new QGraphicsEllipseItem(); ellipseItem->setFlag(QGraphicsItem::ItemIsMovable); QDomElement gElement = gNode.toElement(); ellipseItem->setRect(ellipseElement.attribute("cx").toFloat() - ellipseElement.attribute("rx").toFloat(), ellipseElement.attribute("cy").toFloat() - ellipseElement.attribute("ry").toFloat(), ellipseElement.attribute("rx").toFloat() * 2, ellipseElement.attribute("ry").toFloat() * 2); QColor fillColor(ellipseElement.attribute("style").split(";").at(0).split(":").at(1)); ellipseItem->setBrush(QBrush(fillColor)); QColor strokeColor(gElement.attribute("stroke", "#000000")); strokeColor.setAlphaF(gElement.attribute("stroke-opacity").toFloat()); ellipseItem->setPen(QPen(strokeColor,gElement.attribute("stroke-width", "0").toInt())); ellipsesList.append(ellipseItem); } } file.close(); return ellipsesList; } QRectF ReadSVG::getSizes(const QString filename) { QDomDocument doc; // initialize the QDomDocument object on the stack QFile file(filename); // Open our SVG file // If it did not open or could not transfer content to QDocDocument if (!file.open(QIODevice::ReadOnly) || !doc.setContent(&file)) return QRectF(0,0,200,200); // then return the values for the default scene QDomNodeList list = doc.elementsByTagName("svg"); if(list.length() > 0) { QDomElement svgElement = list.item(0).toElement(); QStringList parameters = svgElement.attribute("viewBox").split(" "); return QRectF(parameters.at(0).toInt(), parameters.at(1).toInt(), parameters.at(2).toInt(), parameters.at(3).toInt()); } return QRectF(0,0,200,200); }
widget.cpp
#include "widget.h" #include "ui_widget.h" #include "readsvg.h" #include <QCursor> #include <QFileDialog> Widget::Widget(QWidget *parent) : QWidget(parent), ui(new Ui::Widget) { ui->setupUi(this); scene = new QGraphicsScene(this); ui->graphicsView->setScene(scene); QBrush redBrush(Qt::red); QBrush blueBrush(Qt::blue); QPen blackPen(Qt::black); blackPen.setWidth(6); elipse = scene->addEllipse(10,10,100,100,blackPen,redBrush); rect = scene->addRect(-10,-10,100,100,blackPen,blueBrush); rect->setFlag(QGraphicsItem::ItemIsMovable, true); } Widget::~Widget() { delete ui; } void Widget::on_pushButton_clicked() { QString fileName= QFileDialog::getSaveFileName(this, "Save image", QCoreApplication::applicationDirPath(), "BMP Files (*.bmp);;JPEG (*.JPEG);;PNG (*.png)" ); if (!fileName.isNull()) { QPixmap pixMap = this->ui->graphicsView->grab(); pixMap.save(fileName); } } void Widget::on_btnSave_clicked() { // Заберём путь к файлу и его имененем, который будем создавать QString newPath = QFileDialog::getSaveFileName(this, trUtf8("Save SVG"), path, tr("SVG files (*.svg)")); if (newPath.isEmpty()) return; path = newPath; QSvgGenerator generator; // Создаём объект генератора файла generator.setFileName(path); // Устанавливаем путь к файлу, куда будет сохраняться векторная графика generator.setSize(QSize(scene->width(), scene->height())); // Устанавливаем размеры рабочей области документа в миллиметрах generator.setViewBox(QRect(0, 0, scene->width(), scene->height())); // Устанавливаем рабочую область в координатах generator.setTitle(trUtf8("SVG Example")); // Титульное название документа generator.setDescription(trUtf8("File created by SVG Example")); // Описание документа // С помощью класса QPainter QPainter painter; painter.begin(&generator); // Устанавливаем устройство/объект в котором будем производить отрисовку scene->render(&painter); // Отрисовываем содержимое сцены с помощью painter в целевое устройство/объект painter.end(); // Заканчиваем отрисовку // По окончанию отрисовки получим векторный файл с содержимым графической сцены } void Widget::on_btnOpen_clicked() { QString newPath = QFileDialog::getOpenFileName(this, trUtf8("Open SVG"), path, tr("SVG files (*.svg)")); if (newPath.isEmpty()) return; path = newPath; scene->clear(); //scene->setSceneRect(ReadSVG::getSizes(path)); // Set the size of the graphic scene // Install the objects on the graphical scene, get them using the getElements /*foreach (QGraphicsRectItem *item, ReadSVG::getElements(path)) { QGraphicsRectItem *rect = item; scene->addItem(rect); }*/ scene->setSceneRect(ReadSVG::getSizes(path)); foreach (QGraphicsEllipseItem *itemE, ReadSVG::getEllipses(path)) { QGraphicsEllipseItem *elips = itemE; scene->addItem(elips); } }
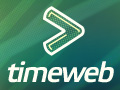
We recommend hosting TIMEWEB
Stable hosting, on which the social network EVILEG is located. For projects on Django we recommend VDS hosting.Do you like it? Share on social networks!
d
- dsfs
- April 26, 2024, 2:56 p.m.
C ++ - Test 004. Pointers, Arrays and Loops
- Result:80points,
- Rating points4
d
- dsfs
- April 26, 2024, 2:35 p.m.
C++ - Test 001. The first program and data types
- Result:73points,
- Rating points1
Last comments
Qt Linux - Lesson 001. Autorun Qt application under Linux как сделать автозапуск для флэтпака, который не даёт создавать файлы в ~/.config - вот это вопрос ))
АК
Qt WinAPI - Lesson 007. Working with ICMP Ping in Qt Без строки #include <QRegularExpressionValidator> в заголовочном файле не работает валидатор.
Анатолий КононенкоFeb. 5, 2024, 12:50 p.m.

EVADec. 25, 2023, 9:30 p.m.
Boost - static linking in CMake project under Windows Сделал всё по-как у вас, но выдаёт ошибку [build] LINK : fatal error LNK1104: не удается открыть файл "libboost_locale-vc142-mt-gd-x64-1_74.lib" Хоть убей, не могу понять в чём дел…
Qt/C++ - Lesson 056. Connecting the Boost library in Qt for MinGW and MSVC compilers Для решения твой проблемы добавь в файл .pro строчку "LIBS += -lws2_32" она решит проблему , лично мне помогло.
Now discuss on the forum

Evgenii LegotckoiMay 3, 2024, 12:07 a.m.

IscanderCheApril 30, 2024, 2:22 p.m.

Павел ДорофеевApril 14, 2024, 12:35 p.m.
Вернуть старое поведение QComboBox, не менять индекс при resetModel Добрый день! У нас много проектов в которых используется QComboBox, в версии 5.5.1, когда модель испускает сигнал resetModel, currentIndex не менялся. В версии 5.15 при resetModel происходит try…
Hello.
Thank you very much sir,my problem solved :) Is this the way to do for other ? I mean polygon,line shape(arrow line)
Yes. Just You need see xml structure of your svg file, tags for figures (polygon, paths, lines and so on) and realize same functions for all figures.
Okay sir :) I'll do so
Good luck.
If You will need some another advice, do not hesitate to ask a new question on this forum.
Okay,Thank you sir :)