A voting site with Django
----------------------------Model---------------------------------------- class Voter(models.Model): gender_type = (('male', 'Male'), ('female', 'Female')) admin = models.OneToOneField(CustomUser, on_delete=models.CASCADE) gender = models.CharField(max_length=10, choices=gender_type) country_region = models.CharField(max_length=255, choices=Countries.choices, default=Countries.Pakistan) phone = PhoneNumberField(blank=True, unique=True) otp = models.CharField(max_length=10, null=True) verified = models.BooleanField(default=False) voted = models.BooleanField(default=False) otp_sent = models.IntegerField(default=0) # Control how many OTPs are sent ip_address = models.GenericIPAddressField(unique=True, default='ABC') def __str__(self): return self.admin.first_name class Position(models.Model): ACTIVE = 0 INACTIVE = 1 status_choice = ((ACTIVE, 'Active'), (INACTIVE, 'Inactive')) name = models.CharField(max_length=50, unique=True) max_vote = models.IntegerField() priority = models.IntegerField() description = models.TextField(null=True, blank=True) status = models.PositiveBigIntegerField(default=0, choices=status_choice) def __str__(self): return self.name class Candidate(models.Model): fullname = models.CharField(max_length=50) photo = models.ImageField(upload_to="candidates") bio = models.TextField() position = models.ForeignKey(Position, on_delete=models.CASCADE, related_name='candidates') def __str__(self): return self.fullname def modify_points(self, added_points): self.points += added_points self.save() class Votes(models.Model): voter = models.ForeignKey(Voter, on_delete=models.CASCADE) position = models.ForeignKey(Position, on_delete=models.CASCADE, related_name='positions') candidate = models.ForeignKey(Candidate, on_delete=models.CASCADE, related_name='votes') class BlockIP(models.Model): voter = models.ForeignKey(Voter, on_delete=models.CASCADE, related_name='ip') position = models.ForeignKey(Position, on_delete=models.CASCADE, related_name='positions_ip', null=True, blank=True) ip_address = models.GenericIPAddressField(default='abc') @receiver(post_save, sender=Voter) def default_to_non_active(sender, instance, created, **kwargs): if created: instance = BlockIP.objects.create(voter=instance, ip_address=instance.ip_address) instance.save() -------------------------------------------- End of model----------------------------------- def submit_ballot(request): if not request.user.is_authenticated: return redirect(reverse('index')) if request.method != 'POST': messages.error(request, "Please, browse the system properly") return redirect(reverse('index')) # Verify if the voter has voted or not voter = request.user.voter blocked = BlockIP.objects.filter(voter=voter) if voter.voted and blocked: messages.error(request, "You have voted already") return redirect(reverse('index')) form = dict(request.POST) form.pop('csrfmiddlewaretoken', None) # Pop CSRF Token form.pop('submit_vote', None) # Pop Submit Button # Ensure at least one vote is selected if len(form.keys()) < 1: messages.error(request, "Please select at least one candidate") return redirect(reverse('index')) positions = Position.objects.all() form_count = 0 for position in positions: max_vote = position.max_vote pos = slugify(position.name) pos_id = position.id if position.max_vote > 1: this_key = pos + "[]" form_position = form.get(this_key) if form_position is None: continue if len(form_position) > max_vote: messages.error(request, "You can only choose " + str(max_vote) + " candidates for " + position.name) return redirect(reverse('index')) else: for form_candidate_id in form_position: form_count += 1 try: candidate = Candidate.objects.get(id=form_candidate_id, position=position) vote = Votes() vote.candidate = candidate vote.voter = voter vote.position = position vote.save() except Exception as e: messages.error( request, "Please, browse the system properly " + str(e)) return redirect(reverse('index')) else: this_key = pos form_position = form.get(this_key) if form_position is None: continue # Max Vote == 1 form_count += 1 try: form_position = form_position[0] candidate = Candidate.objects.get(position=position, id=form_position) vote = Votes() vote.candidate = candidate vote.voter = voter vote.position = position vote.save() except Exception as e: messages.error( request, "Please, browse the system properly " + str(e)) return redirect(reverse('index')) # Count total number of records inserted # Check it viz-a-viz form_count inserted_votes = Votes.objects.filter(voter=voter) if (inserted_votes.count() != form_count): # Delete inserted_votes.delete() messages.error(request, "Please try voting again!") return redirect(reverse('index')) else: # Update Voter profile to voted voter.voted = True voter.save() messages.success(request, "Thanks for voting") return redirect(reverse('index'))
When a poll is create, and a candidate is added to that poll, a voter can vote any candidate, my problem now is if a voter already voted, that voter cannot vote again even when another poll is created, what i want is a voter can still vote in another poll regardless of voting on a poll already
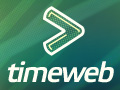
We recommend hosting TIMEWEB
Stable hosting, on which the social network EVILEG is located. For projects on Django we recommend VDS hosting.Do you like it? Share on social networks!
Ua
- Unknown akadamn
- Jan. 24, 2025, 5:14 p.m.
Qt - Test 001. Signals and slots
- Result:84points,
- Rating points4
Ua
- Unknown akadamn
- Jan. 24, 2025, 4:22 p.m.
Qt - Test 001. Signals and slots
- Result:42points,
- Rating points-8
Last comments
ИМ
Django - Tutorial 017. Customize the login page to Django Добрый вечер Евгений! Я сделал себе авторизацию аналогичную вашей, все работает, кроме возврата к предидущей странице. Редеректит всегда на главную, хотя в логах сервера вижу запросы на правильн…
Игорь МаксимовNov. 22, 2024, 9:51 p.m.

Evgenii LegotckoiOct. 31, 2024, 11:37 p.m.
Fb3 file reader on Qt Creator Подскажите как это запустить? Я не шарю в программировании и кодинге. Скачал и установаил Qt, но куча ошибок выдается и не запустить. А очень надо fb3 переконвертировать в html
ИМ
Django - Lesson 064. How to write a Python Markdown extension Приветствую Евгений! У меня вопрос. Можно ли вставлять свои классы в разметку редактора markdown? Допустим имея стандартную разметку: <ul> <li></li> <li></l…
Игорь МаксимовOct. 5, 2024, 4:51 p.m.
QML - Lesson 016. SQLite database and the working with it in QML Qt Здравствуйте, возникает такая проблема (я новичок): ApplicationWindow неизвестный элемент. (М300) для TextField и Button аналогично. Могу предположить, что из-за более новой верси…
Now discuss on the forum
f
Рисование на QGraphicsScene при зажатой кнопке мыши Подскажите, пожалуйста! Как данный класс можно дополнить, чтобы созданные объекты можно было перемещать мышкой по сцене?
firstlunoxodFeb. 15, 2025, 1:46 p.m.

ДмитрийFeb. 3, 2025, 4:24 p.m.
не запускается компьютер!!! Не запускается компьютер (точнее работает блок , но сам монитор вообще жесть)В общем я ничего с интернета не скачивала в последнее время. На компе никаких левых пр…
Нужно запретить перемещение только некоторых итемов, остальные перемещать можно. Вопрос решен. Узнать QModelIndex элемента на который мы перетаскиваем другой элемент, можно с помощью функции indexAt(event->position().toPoint()) представления QTreeViev вызываемой в переопр…
OAuth2.0 через VK, получение email Спасибо большое за помощь и простите за то что отнял время своей невнимательностью.