A voting site with Django
----------------------------Model---------------------------------------- class Voter(models.Model): gender_type = (('male', 'Male'), ('female', 'Female')) admin = models.OneToOneField(CustomUser, on_delete=models.CASCADE) gender = models.CharField(max_length=10, choices=gender_type) country_region = models.CharField(max_length=255, choices=Countries.choices, default=Countries.Pakistan) phone = PhoneNumberField(blank=True, unique=True) otp = models.CharField(max_length=10, null=True) verified = models.BooleanField(default=False) voted = models.BooleanField(default=False) otp_sent = models.IntegerField(default=0) # Control how many OTPs are sent ip_address = models.GenericIPAddressField(unique=True, default='ABC') def __str__(self): return self.admin.first_name class Position(models.Model): ACTIVE = 0 INACTIVE = 1 status_choice = ((ACTIVE, 'Active'), (INACTIVE, 'Inactive')) name = models.CharField(max_length=50, unique=True) max_vote = models.IntegerField() priority = models.IntegerField() description = models.TextField(null=True, blank=True) status = models.PositiveBigIntegerField(default=0, choices=status_choice) def __str__(self): return self.name class Candidate(models.Model): fullname = models.CharField(max_length=50) photo = models.ImageField(upload_to="candidates") bio = models.TextField() position = models.ForeignKey(Position, on_delete=models.CASCADE, related_name='candidates') def __str__(self): return self.fullname def modify_points(self, added_points): self.points += added_points self.save() class Votes(models.Model): voter = models.ForeignKey(Voter, on_delete=models.CASCADE) position = models.ForeignKey(Position, on_delete=models.CASCADE, related_name='positions') candidate = models.ForeignKey(Candidate, on_delete=models.CASCADE, related_name='votes') class BlockIP(models.Model): voter = models.ForeignKey(Voter, on_delete=models.CASCADE, related_name='ip') position = models.ForeignKey(Position, on_delete=models.CASCADE, related_name='positions_ip', null=True, blank=True) ip_address = models.GenericIPAddressField(default='abc') @receiver(post_save, sender=Voter) def default_to_non_active(sender, instance, created, **kwargs): if created: instance = BlockIP.objects.create(voter=instance, ip_address=instance.ip_address) instance.save() -------------------------------------------- End of model----------------------------------- def submit_ballot(request): if not request.user.is_authenticated: return redirect(reverse('index')) if request.method != 'POST': messages.error(request, "Please, browse the system properly") return redirect(reverse('index')) # Verify if the voter has voted or not voter = request.user.voter blocked = BlockIP.objects.filter(voter=voter) if voter.voted and blocked: messages.error(request, "You have voted already") return redirect(reverse('index')) form = dict(request.POST) form.pop('csrfmiddlewaretoken', None) # Pop CSRF Token form.pop('submit_vote', None) # Pop Submit Button # Ensure at least one vote is selected if len(form.keys()) < 1: messages.error(request, "Please select at least one candidate") return redirect(reverse('index')) positions = Position.objects.all() form_count = 0 for position in positions: max_vote = position.max_vote pos = slugify(position.name) pos_id = position.id if position.max_vote > 1: this_key = pos + "[]" form_position = form.get(this_key) if form_position is None: continue if len(form_position) > max_vote: messages.error(request, "You can only choose " + str(max_vote) + " candidates for " + position.name) return redirect(reverse('index')) else: for form_candidate_id in form_position: form_count += 1 try: candidate = Candidate.objects.get(id=form_candidate_id, position=position) vote = Votes() vote.candidate = candidate vote.voter = voter vote.position = position vote.save() except Exception as e: messages.error( request, "Please, browse the system properly " + str(e)) return redirect(reverse('index')) else: this_key = pos form_position = form.get(this_key) if form_position is None: continue # Max Vote == 1 form_count += 1 try: form_position = form_position[0] candidate = Candidate.objects.get(position=position, id=form_position) vote = Votes() vote.candidate = candidate vote.voter = voter vote.position = position vote.save() except Exception as e: messages.error( request, "Please, browse the system properly " + str(e)) return redirect(reverse('index')) # Count total number of records inserted # Check it viz-a-viz form_count inserted_votes = Votes.objects.filter(voter=voter) if (inserted_votes.count() != form_count): # Delete inserted_votes.delete() messages.error(request, "Please try voting again!") return redirect(reverse('index')) else: # Update Voter profile to voted voter.voted = True voter.save() messages.success(request, "Thanks for voting") return redirect(reverse('index'))
When a poll is create, and a candidate is added to that poll, a voter can vote any candidate, my problem now is if a voter already voted, that voter cannot vote again even when another poll is created, what i want is a voter can still vote in another poll regardless of voting on a poll already
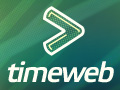
Рекомендуем хостинг TIMEWEB
Стабильный хостинг, на котором располагается социальная сеть EVILEG. Для проектов на Django рекомендуем VDS хостинг.Вам это нравится? Поделитесь в социальных сетях!
Комментарии
Только авторизованные пользователи могут публиковать комментарии.
Пожалуйста, авторизуйтесь или зарегистрируйтесь
Пожалуйста, авторизуйтесь или зарегистрируйтесь
Ua
- Unknown akadamn
- 24 января 2025 г. 7:14
Qt - Тест 001. Сигналы и слоты
- Результат:84баллов,
- Очки рейтинга4
Ua
- Unknown akadamn
- 24 января 2025 г. 6:22
Qt - Тест 001. Сигналы и слоты
- Результат:42баллов,
- Очки рейтинга-8
Последние комментарии
ИМ
Django - Урок 017. Кастомизированная страница авторизации на Django Добрый вечер Евгений! Я сделал себе авторизацию аналогичную вашей, все работает, кроме возврата к предидущей странице. Редеректит всегда на главную, хотя в логах сервера вижу запросы на правильн…
Игорь Максимов22 ноября 2024 г. 11:51

Evgenii Legotckoi31 октября 2024 г. 14:37
Читалка fb3-файлов на Qt Creator Подскажите как это запустить? Я не шарю в программировании и кодинге. Скачал и установаил Qt, но куча ошибок выдается и не запустить. А очень надо fb3 переконвертировать в html
ИМ
Django - Урок 064. Как написать расширение для Python Markdown Приветствую Евгений! У меня вопрос. Можно ли вставлять свои классы в разметку редактора markdown? Допустим имея стандартную разметку: <ul> <li></li> <li></l…
Игорь Максимов5 октября 2024 г. 7:51
QML - Урок 016. База данных SQLite и работа с ней в QML Qt Здравствуйте, возникает такая проблема (я новичок): ApplicationWindow неизвестный элемент. (М300) для TextField и Button аналогично. Могу предположить, что из-за более новой верси…
Сейчас обсуждают на форуме

Дмитрий3 февраля 2025 г. 6:24
не запускается компьютер!!! Не запускается компьютер (точнее работает блок , но сам монитор вообще жесть)В общем я ничего с интернета не скачивала в последнее время. На компе никаких левых пр…
Нужно запретить перемещение только некоторых итемов, остальные перемещать можно. Вопрос решен. Узнать QModelIndex элемента на который мы перетаскиваем другой элемент, можно с помощью функции indexAt(event->position().toPoint()) представления QTreeViev вызываемой в переопр…
OAuth2.0 через VK, получение email Спасибо большое за помощь и простите за то что отнял время своей невнимательностью.

Evgenii Legotckoi24 июня 2024 г. 15:11