G
Gintoki-_-29 августа 2021 г. 0:11
Ошибка undefined reference to
Доброго времени суток!
Полгода назад писал один проект на Qt, получил ошибку и так и не смог разобраться что там не так.
Не могли бы вы глянуть и указать на ошибки?
complex.h
#ifndef COMPLEX_H #define COMPLEX_H #include <QtMath> class Complex { public: qreal real; qreal imag; bool inf; Complex(const qreal &a=0, const qreal &b=0, const bool &c=1); /*Complex(const Complex &a); Complex operator = (const Complex &a);*/ //~Complex(); static qreal norm (const Complex &a); static qreal abs (const Complex &a); static qreal arg (const Complex &a); static Complex conj (const Complex &a); Complex operator - () const; Complex operator + (const Complex &a) const; Complex operator - (const Complex &a) const; Complex operator * (const Complex &a) const; Complex operator / (const Complex &a) const; bool operator == (const Complex &a) const; bool operator != (const Complex &a) const; static Complex exp(const Complex &a); static Complex ln(const Complex &a); static Complex pow (const Complex &a, const Complex &b); static Complex sin(const Complex &a); static Complex cos(const Complex &a); }; #endif
transformation.h
#ifndef TRANSFORMATION_H #define TRANSFORMATION_H #include "complex.h" #include <QVector> //#include "circle.h" class Transformation { private: Complex a; Complex b; Complex c; Complex d; bool e; public: //конструктор Transformation(const Complex &a_1 = {1,0}, const Complex &b_1 = {0, 0}, const Complex &c_1 = {0, 0}, const Complex &d_1 = {1, 0}, const bool &e_1 = 0); Complex Transf(const Complex &G) const; //оператор композиции (последовательное применение) Transformation operator *(const Transformation &t) const; //оператор итерации (последовательное примение одного преобразования несколько раз) Transformation operator ^(const int &k) const; //оператор сравнения, возвращает true если преобразования одинаковы bool operator == (const Transformation &t) const; //оператор сравнения, возвращает true если преобразования различны bool operator != (const Transformation &t) const; //поворот(центр поворота, угол) static Transformation rotate(const Complex &O, const qreal &ang); //static Transformation invers(Circle &G); }; #endif // TRANSFORMATION_H
complex.cpp
#include "complex.h" Complex::Complex(const qreal &a, const qreal &b, const bool &c) : real{a}, imag{b}, inf{c} { } /*Complex::Complex(const Complex &a) : real{a.real}, imag{a.imag}, inf{a.inf} { } Complex Complex::operator =(const Complex &a) { real = a.real; imag = a.imag; inf = a.inf; return {a.real, a.imag, a.inf}; } */ /*Complex::~Complex(){ delete real, imag, inf; }*/ /* { if (a.inf) { out << a.real; if (a.imag==0){ out << "+0i"; } else { if (a.imag > 0) { out << "+"; } out << a.imag << "i"; } } else { out << "∞"; } return out; }*/ qreal Complex::norm(const Complex &a) { return a.real * a.real + a.imag * a.imag; } qreal Complex::abs(const Complex &a) { return qSqrt(Complex::norm(a)); } qreal Complex::arg(const Complex &a) { if (a.real == 0) { return ((a.real > 0) - (a.real < 0)) * qAcos(-1) / 2; } else if ((a.real < 0) && (a.imag > 0)) { return qAcos(-1) + qAtan(a.imag / a.real); } else { return -qAcos(-1) + qAtan(a.imag / a.real); } } Complex Complex::conj(const Complex &a) { return {a.real, -a.imag, a.inf}; } Complex Complex::operator -() const { if (inf) { return {-real,-imag}; } else { return {0, 0, 0}; } } Complex Complex::operator+(const Complex &a) const { if ((inf) && (a.inf)) { return {real + a.real, imag + a.imag}; } else { return {0, 0, 0}; } } Complex Complex::operator-(const Complex &a) const { if ((inf) && (a.inf)) { return {real - a.real, imag - a.imag}; } else { return {0, 0, 0}; } } Complex Complex::operator*(const Complex &a) const { if ((inf) && (a.inf)) { return {real * a.real - imag * a.imag, real * a.imag + imag * a.real}; } else { return {0, 0, 0}; } } Complex Complex::operator/(const Complex &a) const { Complex out; if ((inf) && (a.inf)) { if ((a.real == 0) && (a.imag == 0)) { out={0, 0, 0}; } else { Complex d = *this; Complex r = d * Complex::conj(a); out = {r.real / Complex::norm(a), r.imag / Complex::norm(a)}; } } else if (inf) { out = {0, 0, 1}; } return out; } bool Complex::operator==(const Complex &a) const { if ((real == a.real) && (imag == a.imag) && (inf == a.inf)) { return true; } else { return false; } } bool Complex::operator!=(const Complex &a) const { if ((real - a.real != 0) || (imag - a.imag != 0) || (inf - a.inf != 0)) { return true; } else { return false; } } Complex Complex::exp(const Complex &a) { if (a.inf) { return {qExp(a.real) * qCos(a.imag), qExp(a.real) * qSin(a.imag)}; } else { return {0, 0, 0}; } } Complex Complex::ln(const Complex &a) { if ((a.real==0)&&(a.imag==0)) { return {0,0,0}; } else { return {qLn(Complex::abs(a)), qAcos(a.real / Complex::abs(a))}; } } Complex Complex::pow(const Complex &a, const Complex &b) { return (Complex::exp(Complex::ln(a) * b)); } Complex Complex::sin(const Complex &a) { return (Complex::exp(Complex(0, 1) * a) - Complex::exp(Complex(0, -1) * a)) / Complex(0, 2); } Complex Complex::cos(const Complex &a) { return (Complex::exp(Complex(0, 1) * a) + Complex::exp(Complex(0, -1) * a)) / Complex(2, 0); }
transformation.cpp
#include "transformation.h" Transformation::Transformation(const Complex &a_1, const Complex &b_1, const Complex &c_1, const Complex &d_1, const bool &e_1) : a{a_1}, b{b_1}, c{c_1}, d{d_1}, e{e_1} { } Complex Transformation::Transf(const Complex &G) const { if (e) { //e=1 nesobstv e=0 sobstv return (a * Complex::conj(G) + b)/(c * Complex::conj(G) + d); } else { return (a * G + b)/(c * G + d); } } Transformation Transformation::operator *(const Transformation &t) const { if(e){ return { ((a * Complex::conj(t.a)) + (b * Complex::conj(t.c))), ((a * Complex::conj(t.b)) + (b * Complex::conj(t.d))), ((c * Complex::conj(t.a)) + (d * Complex::conj(t.c))), ((c * Complex::conj(t.b)) + (d * Complex::conj(t.d))), (e != t.e) }; } else { return { ((a * t.a) + (b * t.c)), ((a * t.b) + (b * t.d)), ((c * t.a) + (d * t.c)), ((c * t.b) + (d * t.d)), (e != t.e) }; } } Transformation Transformation::operator ^(const int &k) const { int l=k; Transformation t; Transformation f={a, b, c, d, e}; Transformation g={d, -b, -c, a, e}; if(k>0){ while(l--){ t=t*f; } } else { while(l++){ t=t*g; } } return t; } bool Transformation::operator == (const Transformation &t) const { if((a==t.a)&&(b==t.b)&&(c==t.c)&&(d==t.d)&&(e==t.e)){ return 0; } else { return 1; } } bool Transformation::operator != (const Transformation &t) const{ if((a!=t.a)||(b!=t.b)||(c!=t.c)||(d!=t.d)||(e!=t.e)){ return 0; } else { return 1; } } Transformation Transformation::rotate(const Complex &O, const qreal &ang) { Complex g = {qreal(cos(ang)), qreal(sin(ang))}; return { g, O - O * g, {0, 0}, {1, 0}, 0 }; } /*Transformation Transformation::invers(Circle &G) { QVector<Complex> F = G.getCenter_and_Radius(); if (F.at(0) == Complex(2,0)) { QVector<Complex> K=G.equation(); return { K.at(2)/K.at(0), -K.at(3)/K.at(0), Complex(1,0), -Complex::conj(K.at(2)/K.at(0)), 1 }; } else { Complex g = G.A - G.B; return { g, G.B * Complex::conj(G.A) - G.A * Complex::conj(G.B), 0, Complex::conj(g), 1 }; } }*/ /*auto fixp() { a=initializatoin if (e){ if(c==Co_Z){ } else { } } else { if(c==Co_Z){ if(a==Co_1){ return {Co_1/Co_i}; } else { return {b/(Co_1-a),Co_1/Co_i}; } } else { } } return {a}; }*/
pro файл
QT += core gui greaterThan(QT_MAJOR_VERSION, 4): QT += widgets CONFIG += c++14 # The following define makes your compiler emit warnings if you use # any Qt feature that has been marked deprecated (the exact warnings # depend on your compiler). Please consult the documentation of the # deprecated API in order to know how to port your code away from it. DEFINES += QT_DEPRECATED_WARNINGS # You can also make your code fail to compile if it uses deprecated APIs. # In order to do so, uncomment the following line. # You can also select to disable deprecated APIs only up to a certain version of Qt. #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 SOURCES += \ circle.cpp \ complex.cpp \ main.cpp \ mainwindow.cpp \ simplemenu.cpp \ transformation.cpp \ view.cpp HEADERS += \ circle.h \ complex.h \ mainwindow.h \ simplemenu.h \ transformation.h \ view.h FORMS += \ mainwindow.ui \ simplemenu.ui # Default rules for deployment. qnx: target.path = /tmp/$${TARGET}/bin else: unix:!android: target.path = /opt/$${TARGET}/bin !isEmpty(target.path): INSTALLS += target
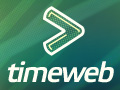
Рекомендуем хостинг TIMEWEB
Стабильный хостинг, на котором располагается социальная сеть EVILEG. Для проектов на Django рекомендуем VDS хостинг.Вам это нравится? Поделитесь в социальных сетях!
Комментарии
Только авторизованные пользователи могут публиковать комментарии.
Пожалуйста, авторизуйтесь или зарегистрируйтесь
Пожалуйста, авторизуйтесь или зарегистрируйтесь
Ua
- Unknown akadamn
- 24 января 2025 г. 17:14
Qt - Тест 001. Сигналы и слоты
- Результат:84баллов,
- Очки рейтинга4
Ua
- Unknown akadamn
- 24 января 2025 г. 16:22
Qt - Тест 001. Сигналы и слоты
- Результат:42баллов,
- Очки рейтинга-8
Последние комментарии
ИМ
Django - Урок 017. Кастомизированная страница авторизации на Django Добрый вечер Евгений! Я сделал себе авторизацию аналогичную вашей, все работает, кроме возврата к предидущей странице. Редеректит всегда на главную, хотя в логах сервера вижу запросы на правильн…
Игорь Максимов22 ноября 2024 г. 21:51

Evgenii Legotckoi31 октября 2024 г. 23:37
Читалка fb3-файлов на Qt Creator Подскажите как это запустить? Я не шарю в программировании и кодинге. Скачал и установаил Qt, но куча ошибок выдается и не запустить. А очень надо fb3 переконвертировать в html
ИМ
Django - Урок 064. Как написать расширение для Python Markdown Приветствую Евгений! У меня вопрос. Можно ли вставлять свои классы в разметку редактора markdown? Допустим имея стандартную разметку: <ul> <li></li> <li></l…
Игорь Максимов5 октября 2024 г. 16:51
QML - Урок 016. База данных SQLite и работа с ней в QML Qt Здравствуйте, возникает такая проблема (я новичок): ApplicationWindow неизвестный элемент. (М300) для TextField и Button аналогично. Могу предположить, что из-за более новой верси…
Сейчас обсуждают на форуме
f
Рисование на QGraphicsScene при зажатой кнопке мыши Подскажите, пожалуйста! Как данный класс можно дополнить, чтобы созданные объекты можно было перемещать мышкой по сцене?
firstlunoxod15 февраля 2025 г. 13:46

Дмитрий3 февраля 2025 г. 16:24
не запускается компьютер!!! Не запускается компьютер (точнее работает блок , но сам монитор вообще жесть)В общем я ничего с интернета не скачивала в последнее время. На компе никаких левых пр…
Нужно запретить перемещение только некоторых итемов, остальные перемещать можно. Вопрос решен. Узнать QModelIndex элемента на который мы перетаскиваем другой элемент, можно с помощью функции indexAt(event->position().toPoint()) представления QTreeViev вызываемой в переопр…
OAuth2.0 через VK, получение email Спасибо большое за помощь и простите за то что отнял время своей невнимательностью.
Похоже, ошибка была в pro файле: в 1 строке лишний отступ? (Можете рассказать про синтаксис pro файла?). Пойду проверять работает ли всё
Маловероятно, скорее всего нужно было перезапустить qmake после создания файла.